mirror of https://github.com/dapr/java-sdk.git
Modify the pubsub example to have custom span. (#538)
This commit is contained in:
parent
7467575a97
commit
dfd0d5c7af
|
@ -0,0 +1,82 @@
|
|||
/*
|
||||
* Copyright (c) Microsoft Corporation and Dapr Contributors.
|
||||
* Licensed under the MIT License.
|
||||
*/
|
||||
|
||||
package io.dapr.examples.pubsub.http;
|
||||
|
||||
import io.dapr.client.DaprClient;
|
||||
import io.dapr.client.DaprClientBuilder;
|
||||
import io.dapr.examples.OpenTelemetryConfig;
|
||||
import io.opentelemetry.api.OpenTelemetry;
|
||||
import io.opentelemetry.api.trace.Span;
|
||||
import io.opentelemetry.api.trace.Tracer;
|
||||
import io.opentelemetry.context.Scope;
|
||||
import io.opentelemetry.sdk.OpenTelemetrySdk;
|
||||
|
||||
import static io.dapr.examples.OpenTelemetryConfig.getReactorContext;
|
||||
|
||||
/**
|
||||
* Message publisher.
|
||||
* 1. Build and install jars:
|
||||
* mvn clean install
|
||||
* 2. cd [repo root]/examples
|
||||
* 3. Run the program:
|
||||
* dapr run --components-path ./components/pubsub --app-id publisher_tracing -- \
|
||||
* java -jar target/dapr-java-sdk-examples-exec.jar io.dapr.examples.pubsub.http.PublisherWithTracing
|
||||
*/
|
||||
public class PublisherWithTracing {
|
||||
|
||||
//Number of messages to be sent.
|
||||
private static final int NUM_MESSAGES = 10;
|
||||
|
||||
//The title of the topic to be used for publishing
|
||||
private static final String TOPIC_NAME = "testingtopic";
|
||||
|
||||
//The name of the pubsub
|
||||
private static final String PUBSUB_NAME = "messagebus";
|
||||
|
||||
/**
|
||||
* This is the entry point of the publisher app example.
|
||||
*
|
||||
* @param args Args, unused.
|
||||
* @throws Exception A startup Exception.
|
||||
*/
|
||||
public static void main(String[] args) throws Exception {
|
||||
OpenTelemetry openTelemetry = OpenTelemetryConfig.createOpenTelemetry();
|
||||
Tracer tracer = openTelemetry.getTracer(PublisherWithTracing.class.getCanonicalName());
|
||||
Span span = tracer.spanBuilder("Publisher's Main").setSpanKind(Span.Kind.CLIENT).startSpan();
|
||||
|
||||
try (DaprClient client = new DaprClientBuilder().build()) {
|
||||
try (Scope scope = span.makeCurrent()) {
|
||||
for (int i = 0; i < NUM_MESSAGES; i++) {
|
||||
String message = String.format("This is message #%d", i);
|
||||
// Publishing messages, notice the use of subscriberContext() for tracing.
|
||||
client.publishEvent(
|
||||
PUBSUB_NAME,
|
||||
TOPIC_NAME,
|
||||
message).subscriberContext(getReactorContext()).block();
|
||||
System.out.println("Published message: " + message);
|
||||
|
||||
try {
|
||||
Thread.sleep((long) (1000 * Math.random()));
|
||||
} catch (InterruptedException e) {
|
||||
e.printStackTrace();
|
||||
Thread.currentThread().interrupt();
|
||||
return;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// Close the span.
|
||||
span.end();
|
||||
|
||||
// Shutdown the OpenTelemetry tracer.
|
||||
OpenTelemetrySdk.getGlobalTracerManagement().shutdown();
|
||||
|
||||
// This is an example, so for simplicity we are just exiting here.
|
||||
// Normally a dapr app would be a web service and not exit main.
|
||||
System.out.println("Done.");
|
||||
}
|
||||
}
|
||||
}
|
|
@ -238,6 +238,41 @@ Once running, the Subscriber should print the output as follows:
|
|||
|
||||
Messages have been retrieved from the topic.
|
||||
|
||||
### Tracing
|
||||
|
||||
Dapr handles tracing in PubSub automatically. Open Zipkin on [http://localhost:9411/zipkin](http://localhost:9411/zipkin). You should see a screen like the one below:
|
||||
|
||||
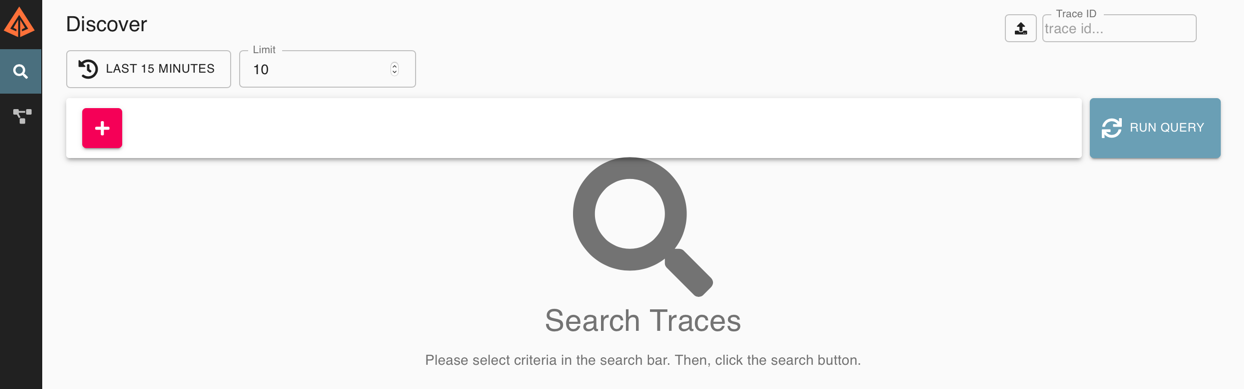
|
||||
|
||||
Click on the search icon to see the latest query results. You should see a tracing diagram similar to the one below:
|
||||
|
||||
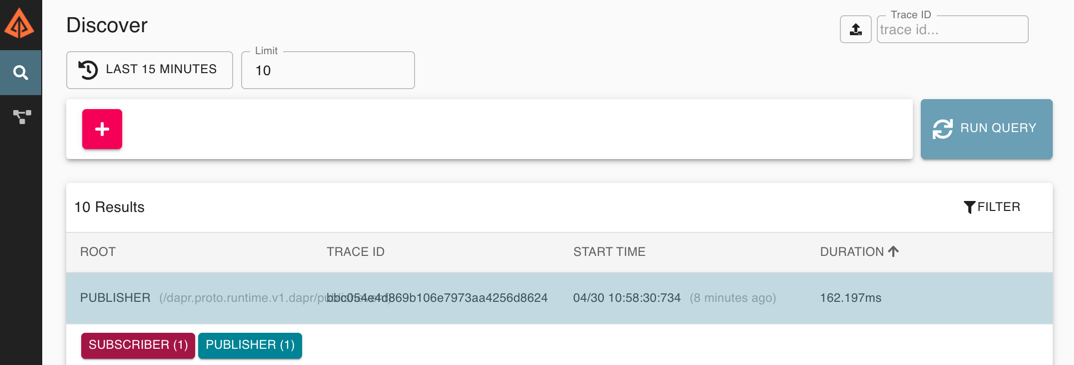
|
||||
|
||||
Once you click on the tracing event, you will see the details of the call stack starting in the client and then showing the service API calls right below.
|
||||
|
||||
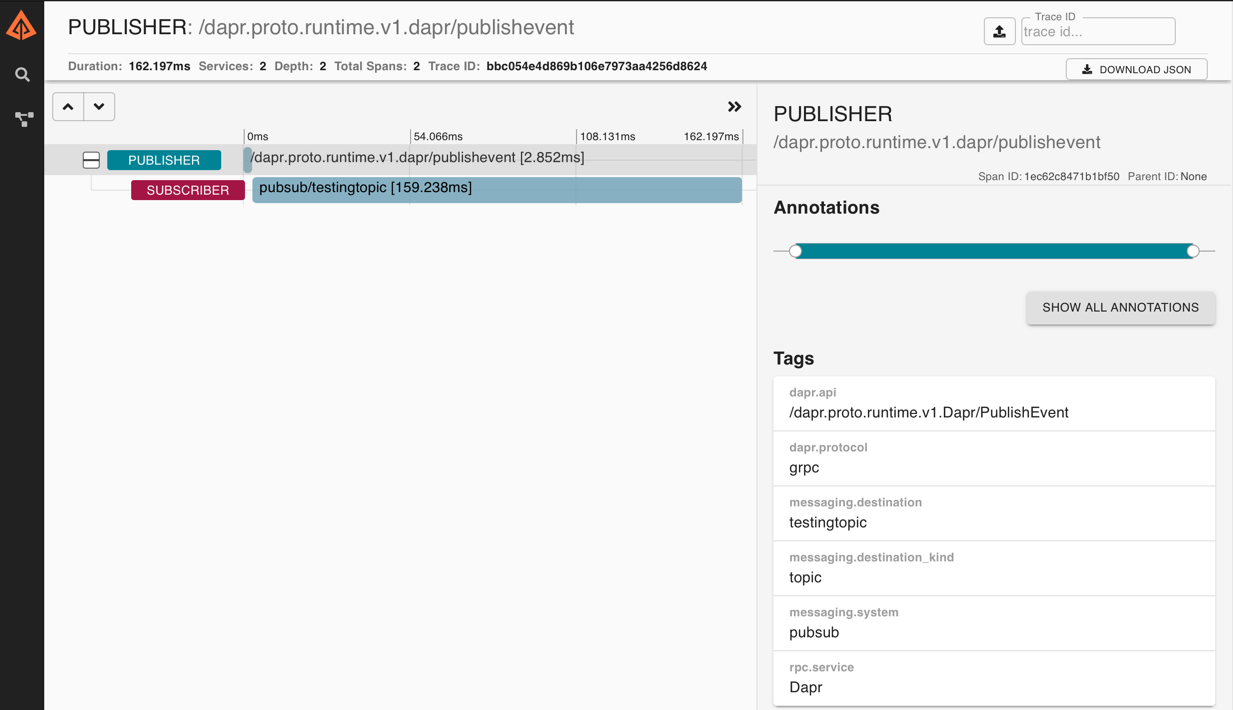
|
||||
|
||||
If you would like to add a tracing span as a parent of the span created by Dapr, change the publisher to handle that. See `PublisherWithTracing.java` to see the difference and run it with:
|
||||
|
||||
<!-- STEP
|
||||
name: Run Publisher
|
||||
expected_stdout_lines:
|
||||
- '== APP == Published message: This is message #0'
|
||||
- '== APP == Published message: This is message #1'
|
||||
background: true
|
||||
sleep: 15
|
||||
-->
|
||||
|
||||
```bash
|
||||
dapr run --components-path ./components/pubsub --app-id publisher_tracing -- java -jar target/dapr-java-sdk-examples-exec.jar io.dapr.examples.pubsub.http.PublisherWithTracing
|
||||
```
|
||||
|
||||
<!-- END_STEP -->
|
||||
|
||||
Now, repeat the search on Zipkin website. All the publisher and subscriber spans are under the same parent span, like in the screen below:
|
||||
|
||||
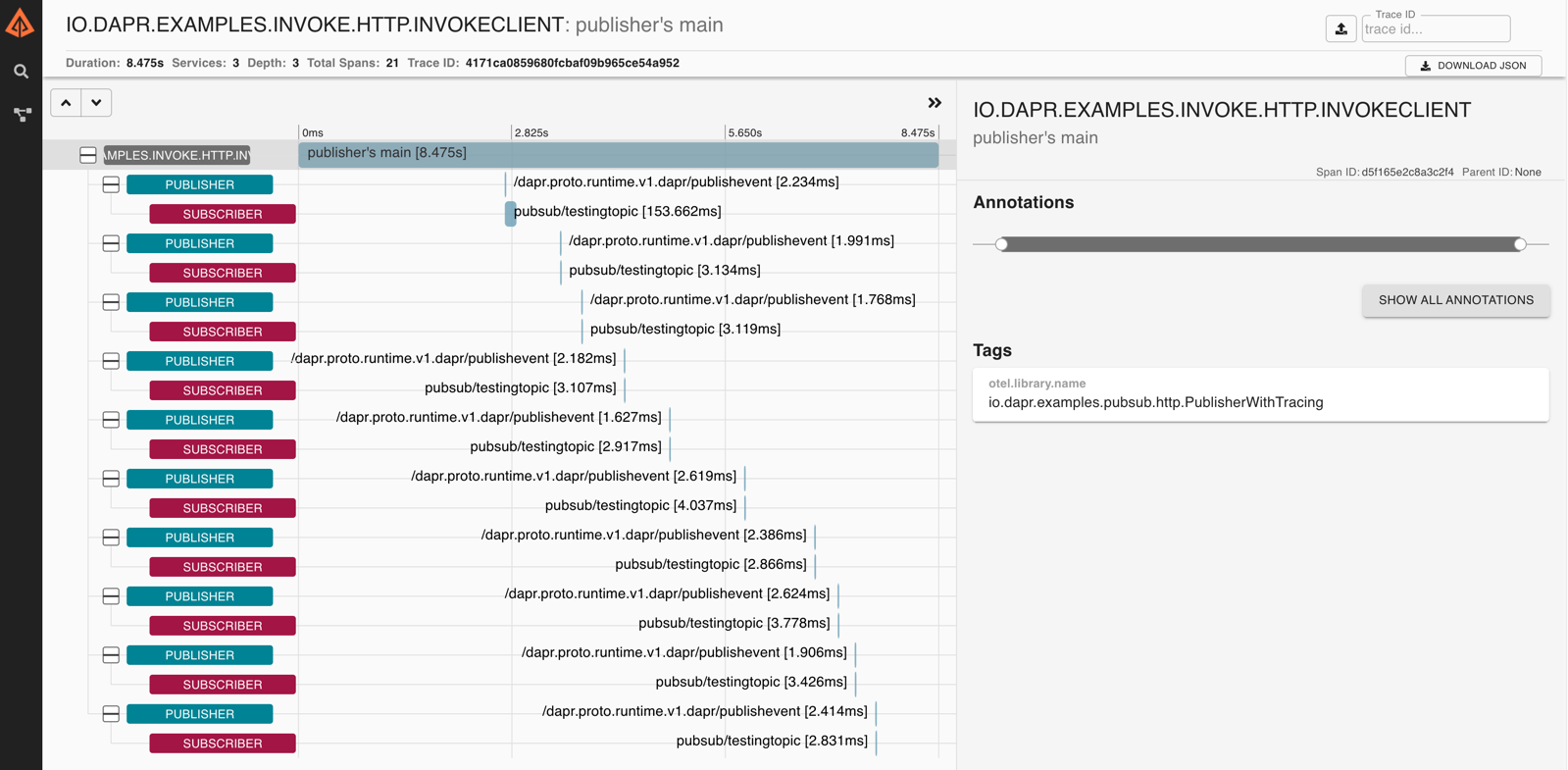
|
||||
|
||||
### Message expiration (Optional)
|
||||
|
||||
Optionally, you can see how Dapr can automatically drop expired messages on behalf of the subscriber.
|
||||
|
|
Binary file not shown.
After Width: | Height: | Size: 258 KiB |
Binary file not shown.
After Width: | Height: | Size: 99 KiB |
Binary file not shown.
After Width: | Height: | Size: 39 KiB |
Binary file not shown.
After Width: | Height: | Size: 46 KiB |
Loading…
Reference in New Issue